React.js Nested Nav BarReact.js flood game implementationReact.js components for a golf appManipulate original DOM in React.jsCountdown timer in React.jsReact.JS functional paradigmSimple webpage on React.jsReact.js defaultMemoize as containerCreating table using React.jsFiltered table in React.jsRecursive react.js navigation menu
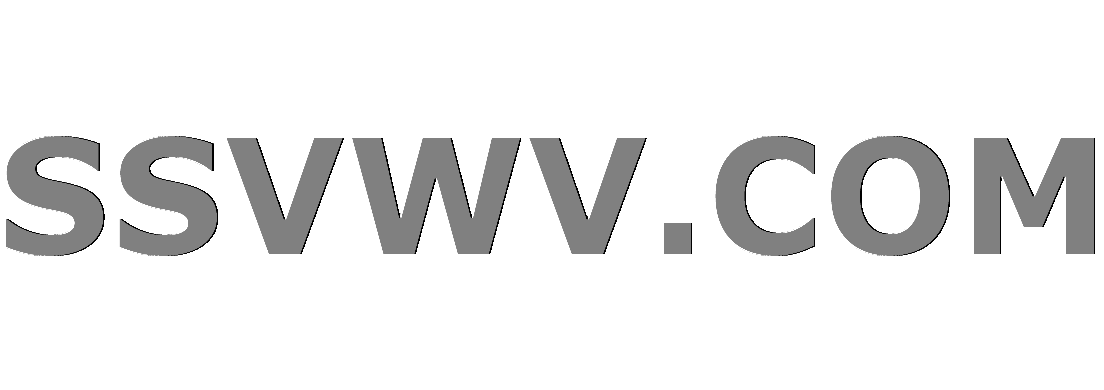
Multi tool use
System.QueryException unexpected token
Open a doc from terminal, but not by its name
How much character growth crosses the line into breaking the character
Pre-mixing cryogenic fuels and using only one fuel tank
Unexpected behavior of the procedure `Area` on the object 'Polygon'
Why does a simple loop result in ASYNC_NETWORK_IO waits?
Mimic lecturing on blackboard, facing audience
Does an advisor owe his/her student anything? Will an advisor keep a PhD student only out of pity?
Terse Method to Swap Lowest for Highest?
A binary search solution to 3Sum
Mixing PEX brands
Recommended PCB layout understanding - ADM2572 datasheet
Is there a RAID 0 Equivalent for RAM?
What does "Scientists rise up against statistical significance" mean? (Comment in Nature)
Invalid date error by date command
Does the UK parliament need to pass secondary legislation to accept the Article 50 extension
Calculating total slots
Why did the EU agree to delay the Brexit deadline?
User Story breakdown - Technical Task + User Feature
What to do when eye contact makes your subordinate uncomfortable?
Electoral considerations aside, what are potential benefits, for the US, of policy changes proposed by the tweet recognizing Golan annexation?
Limits and Infinite Integration by Parts
Why is it that I can sometimes guess the next note?
How to explain what's wrong with this application of the chain rule?
React.js Nested Nav Bar
React.js flood game implementationReact.js components for a golf appManipulate original DOM in React.jsCountdown timer in React.jsReact.JS functional paradigmSimple webpage on React.jsReact.js defaultMemoize as containerCreating table using React.jsFiltered table in React.jsRecursive react.js navigation menu
$begingroup$
This was written for a coding challenge for a company I recently starting working for. I'm looking for any suggestions on how to clean up the code, as well as any issues anyone thinks may occur as it is written now. Fairly new to React.js, always looking to improve.
Currently the data is passed through the app component where I render the Nav Container (functional stateless component). The Nav container creates the classNames and passes data + classNames to the NestedNav that I've put below.
It started to feel a little sloppy while mapping twice below the render, not sure if that's a problem?
The data object example shows how I modeled my data. This example has a parent with children that also have children.
- The parentClass is for the outer parent.
- The nestedParentClass is for the children of the outer parent.
- The childClass is reserved for any children of the nestedParent.
export const data = [
id: 1,
icon: <FontAwesomeIcon className='i' icon=faHeartbeat />,
title: 'SAFETY',
children: [
title: 'Reporting', children: [
id: 11, title: 'I-21 Injury Reporting', url: '/safety/report',
id: 12, title: 'ASAP Reporting', url: '/safety/asap-report',
id: 13, title: 'General ASAP Information', url: '/safety/general',
id: 14, title: 'Flight Attendant Incident Report', url: '/safety/flight-attendant-incident',
],
title: 'Agriculture and Customs', children: [
id: 15, title: 'Product Safety Data Search', url: '/safety/data-search'],
id: 16, title: 'Known Crewmember', url: '/safety/known-cremember',
id: 17, title: 'Product Safety Data Search', url: '/safety/data-search',
],
,
]
class NestedNav extends Component
constructor(props)
super(props)
this.state =
selected: '',
nestSelect: '',
children: [],
active: '',
handleClick = (title) =>
this.setState(
selected: '',
nestSelect: '',
children: [],
active: '',
)
render()
const data, parentClass, nestedParentClass = this.props
const renderedChildElements = this.state.children
const active = this.state.active === 'true' ? 'true' : ''
const mappedChildren = (child, title) =>
let childElement
if(child)
childElement = child.map((child, i) => <li
key=i
id=child.id
className=nestedParentClass + (this.state.nestSelect === child.title ? 'nest-selected' : '')
url=child.url><Link to='/'>child.title</Link>
child.children ?
<FontAwesomeIcon
onClick=() => mappedChildren(child.children, this.state.select)
className='i button'
icon=faArrowCircleDown /> : null
</li>)
this.setState(
selected: title,
children: childElement,
active: 'true',
)
return ''
const navListItems = data.map((collection, i) => <li
key=i
id=collection.id
className=parentClass + (this.state.selected === collection.title ? 'selected' : '')
url=collection.url><i onClick=this.handleClick>collection.icon</i><Link to='/'><span>collection.title</span></Link>
collection.children ?
<FontAwesomeIcon
onClick=() => mappedChildren(collection.children, collection.title)
className='i button'
icon=faArrowCircleRight /> : null
</li>)
return (
<div className='nested-nav'>
<div className='container-two-' + active>
<h2>this.state.selected</h2>
this.state.children
</div>
<div className='container-one'>navListItems</div>
</div>
)
export default NestedNav
javascript programming-challenge recursion functional-programming react.js
$endgroup$
bumped to the homepage by Community♦ 12 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
$begingroup$
This was written for a coding challenge for a company I recently starting working for. I'm looking for any suggestions on how to clean up the code, as well as any issues anyone thinks may occur as it is written now. Fairly new to React.js, always looking to improve.
Currently the data is passed through the app component where I render the Nav Container (functional stateless component). The Nav container creates the classNames and passes data + classNames to the NestedNav that I've put below.
It started to feel a little sloppy while mapping twice below the render, not sure if that's a problem?
The data object example shows how I modeled my data. This example has a parent with children that also have children.
- The parentClass is for the outer parent.
- The nestedParentClass is for the children of the outer parent.
- The childClass is reserved for any children of the nestedParent.
export const data = [
id: 1,
icon: <FontAwesomeIcon className='i' icon=faHeartbeat />,
title: 'SAFETY',
children: [
title: 'Reporting', children: [
id: 11, title: 'I-21 Injury Reporting', url: '/safety/report',
id: 12, title: 'ASAP Reporting', url: '/safety/asap-report',
id: 13, title: 'General ASAP Information', url: '/safety/general',
id: 14, title: 'Flight Attendant Incident Report', url: '/safety/flight-attendant-incident',
],
title: 'Agriculture and Customs', children: [
id: 15, title: 'Product Safety Data Search', url: '/safety/data-search'],
id: 16, title: 'Known Crewmember', url: '/safety/known-cremember',
id: 17, title: 'Product Safety Data Search', url: '/safety/data-search',
],
,
]
class NestedNav extends Component
constructor(props)
super(props)
this.state =
selected: '',
nestSelect: '',
children: [],
active: '',
handleClick = (title) =>
this.setState(
selected: '',
nestSelect: '',
children: [],
active: '',
)
render()
const data, parentClass, nestedParentClass = this.props
const renderedChildElements = this.state.children
const active = this.state.active === 'true' ? 'true' : ''
const mappedChildren = (child, title) =>
let childElement
if(child)
childElement = child.map((child, i) => <li
key=i
id=child.id
className=nestedParentClass + (this.state.nestSelect === child.title ? 'nest-selected' : '')
url=child.url><Link to='/'>child.title</Link>
child.children ?
<FontAwesomeIcon
onClick=() => mappedChildren(child.children, this.state.select)
className='i button'
icon=faArrowCircleDown /> : null
</li>)
this.setState(
selected: title,
children: childElement,
active: 'true',
)
return ''
const navListItems = data.map((collection, i) => <li
key=i
id=collection.id
className=parentClass + (this.state.selected === collection.title ? 'selected' : '')
url=collection.url><i onClick=this.handleClick>collection.icon</i><Link to='/'><span>collection.title</span></Link>
collection.children ?
<FontAwesomeIcon
onClick=() => mappedChildren(collection.children, collection.title)
className='i button'
icon=faArrowCircleRight /> : null
</li>)
return (
<div className='nested-nav'>
<div className='container-two-' + active>
<h2>this.state.selected</h2>
this.state.children
</div>
<div className='container-one'>navListItems</div>
</div>
)
export default NestedNav
javascript programming-challenge recursion functional-programming react.js
$endgroup$
bumped to the homepage by Community♦ 12 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
$begingroup$
This was written for a coding challenge for a company I recently starting working for. I'm looking for any suggestions on how to clean up the code, as well as any issues anyone thinks may occur as it is written now. Fairly new to React.js, always looking to improve.
Currently the data is passed through the app component where I render the Nav Container (functional stateless component). The Nav container creates the classNames and passes data + classNames to the NestedNav that I've put below.
It started to feel a little sloppy while mapping twice below the render, not sure if that's a problem?
The data object example shows how I modeled my data. This example has a parent with children that also have children.
- The parentClass is for the outer parent.
- The nestedParentClass is for the children of the outer parent.
- The childClass is reserved for any children of the nestedParent.
export const data = [
id: 1,
icon: <FontAwesomeIcon className='i' icon=faHeartbeat />,
title: 'SAFETY',
children: [
title: 'Reporting', children: [
id: 11, title: 'I-21 Injury Reporting', url: '/safety/report',
id: 12, title: 'ASAP Reporting', url: '/safety/asap-report',
id: 13, title: 'General ASAP Information', url: '/safety/general',
id: 14, title: 'Flight Attendant Incident Report', url: '/safety/flight-attendant-incident',
],
title: 'Agriculture and Customs', children: [
id: 15, title: 'Product Safety Data Search', url: '/safety/data-search'],
id: 16, title: 'Known Crewmember', url: '/safety/known-cremember',
id: 17, title: 'Product Safety Data Search', url: '/safety/data-search',
],
,
]
class NestedNav extends Component
constructor(props)
super(props)
this.state =
selected: '',
nestSelect: '',
children: [],
active: '',
handleClick = (title) =>
this.setState(
selected: '',
nestSelect: '',
children: [],
active: '',
)
render()
const data, parentClass, nestedParentClass = this.props
const renderedChildElements = this.state.children
const active = this.state.active === 'true' ? 'true' : ''
const mappedChildren = (child, title) =>
let childElement
if(child)
childElement = child.map((child, i) => <li
key=i
id=child.id
className=nestedParentClass + (this.state.nestSelect === child.title ? 'nest-selected' : '')
url=child.url><Link to='/'>child.title</Link>
child.children ?
<FontAwesomeIcon
onClick=() => mappedChildren(child.children, this.state.select)
className='i button'
icon=faArrowCircleDown /> : null
</li>)
this.setState(
selected: title,
children: childElement,
active: 'true',
)
return ''
const navListItems = data.map((collection, i) => <li
key=i
id=collection.id
className=parentClass + (this.state.selected === collection.title ? 'selected' : '')
url=collection.url><i onClick=this.handleClick>collection.icon</i><Link to='/'><span>collection.title</span></Link>
collection.children ?
<FontAwesomeIcon
onClick=() => mappedChildren(collection.children, collection.title)
className='i button'
icon=faArrowCircleRight /> : null
</li>)
return (
<div className='nested-nav'>
<div className='container-two-' + active>
<h2>this.state.selected</h2>
this.state.children
</div>
<div className='container-one'>navListItems</div>
</div>
)
export default NestedNav
javascript programming-challenge recursion functional-programming react.js
$endgroup$
This was written for a coding challenge for a company I recently starting working for. I'm looking for any suggestions on how to clean up the code, as well as any issues anyone thinks may occur as it is written now. Fairly new to React.js, always looking to improve.
Currently the data is passed through the app component where I render the Nav Container (functional stateless component). The Nav container creates the classNames and passes data + classNames to the NestedNav that I've put below.
It started to feel a little sloppy while mapping twice below the render, not sure if that's a problem?
The data object example shows how I modeled my data. This example has a parent with children that also have children.
- The parentClass is for the outer parent.
- The nestedParentClass is for the children of the outer parent.
- The childClass is reserved for any children of the nestedParent.
export const data = [
id: 1,
icon: <FontAwesomeIcon className='i' icon=faHeartbeat />,
title: 'SAFETY',
children: [
title: 'Reporting', children: [
id: 11, title: 'I-21 Injury Reporting', url: '/safety/report',
id: 12, title: 'ASAP Reporting', url: '/safety/asap-report',
id: 13, title: 'General ASAP Information', url: '/safety/general',
id: 14, title: 'Flight Attendant Incident Report', url: '/safety/flight-attendant-incident',
],
title: 'Agriculture and Customs', children: [
id: 15, title: 'Product Safety Data Search', url: '/safety/data-search'],
id: 16, title: 'Known Crewmember', url: '/safety/known-cremember',
id: 17, title: 'Product Safety Data Search', url: '/safety/data-search',
],
,
]
class NestedNav extends Component
constructor(props)
super(props)
this.state =
selected: '',
nestSelect: '',
children: [],
active: '',
handleClick = (title) =>
this.setState(
selected: '',
nestSelect: '',
children: [],
active: '',
)
render()
const data, parentClass, nestedParentClass = this.props
const renderedChildElements = this.state.children
const active = this.state.active === 'true' ? 'true' : ''
const mappedChildren = (child, title) =>
let childElement
if(child)
childElement = child.map((child, i) => <li
key=i
id=child.id
className=nestedParentClass + (this.state.nestSelect === child.title ? 'nest-selected' : '')
url=child.url><Link to='/'>child.title</Link>
child.children ?
<FontAwesomeIcon
onClick=() => mappedChildren(child.children, this.state.select)
className='i button'
icon=faArrowCircleDown /> : null
</li>)
this.setState(
selected: title,
children: childElement,
active: 'true',
)
return ''
const navListItems = data.map((collection, i) => <li
key=i
id=collection.id
className=parentClass + (this.state.selected === collection.title ? 'selected' : '')
url=collection.url><i onClick=this.handleClick>collection.icon</i><Link to='/'><span>collection.title</span></Link>
collection.children ?
<FontAwesomeIcon
onClick=() => mappedChildren(collection.children, collection.title)
className='i button'
icon=faArrowCircleRight /> : null
</li>)
return (
<div className='nested-nav'>
<div className='container-two-' + active>
<h2>this.state.selected</h2>
this.state.children
</div>
<div className='container-one'>navListItems</div>
</div>
)
export default NestedNav
javascript programming-challenge recursion functional-programming react.js
javascript programming-challenge recursion functional-programming react.js
edited Feb 18 at 22:39


Stephen Rauch
3,77061630
3,77061630
asked Feb 18 at 21:18
PnskPnsk
162
162
bumped to the homepage by Community♦ 12 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
bumped to the homepage by Community♦ 12 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
$begingroup$
it's clear and readable but i would like to point out some things :
Avoid using functions inside Render()
A function in the render method will be created each render which is a
slight performance hit. t's also messy if you put them in the render, which is a much > bigger reason
Use the child.id
instead of key
as index
when you map
through an array in render()
because Index as a key is an anti-pattern.
Keep your code as DRY as possible, the initialState can be an object outside the class instead of writing it twice or more, especially if it's a big object.
Optional : use Destructuring assignment to indicate which keys you will use in the function.
const initialState =
selected: '',
nestSelect: '',
children: [],
active: '',
class NestedNav extends Component
constructor(props)
super(props)
this.state = initialState
handleClick = title =>
this.setState( ...initialState )
mappedChildren = (child, selectedTitle) =>
const nestedParentClass = this.props
let childElement
if (child)
childElement = child.map(( id, title, children, url ) => <li
key=id
id=id
className=nestedParentClass + (this.state.nestSelect === title ? 'nest-selected' : '')
url=url><Link to='/'>title</Link>
children ?
<FontAwesomeIcon
onClick=() => this.mappedChildren(children, this.state.select)
className='i button'
icon=faArrowCircleDown /> : null
</li>)
this.setState(
selected: selectedTitle,
children: childElement,
active: 'true',
)
return ''
navListItems = data => data.map(( id, url, children, title, icon ) =>
const parentClass = this.props
return (<li
key=id
id=id
className=parentClass + (this.state.selected === title ? 'selected' : '')
url=url><i onClick=this.handleClick>icon</i><Link to='/'><span>title</span></Link>
children ?
<FontAwesomeIcon
onClick=() => this.mappedChildren(children, title)
className='i button'
icon=faArrowCircleRight /> : null
</li>)
);
render()
const data = this.props
const active = this.state.active === 'true' ? 'true' : ''
return (
<div className='nested-nav'>
<div className='container-two-' + active>
<h2>this.state.selected</h2>
this.state.children
</div>
<div className='container-one'>this.navListItems(data)</div>
</div>
)
export default NestedNav
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
return StackExchange.using("mathjaxEditing", function ()
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix)
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
);
);
, "mathjax-editing");
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "196"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
var $window = $(window),
onScroll = function(e)
var $elem = $('.new-login-left'),
docViewTop = $window.scrollTop(),
docViewBottom = docViewTop + $window.height(),
elemTop = $elem.offset().top,
elemBottom = elemTop + $elem.height();
if ((docViewTop elemBottom))
StackExchange.using('gps', function() StackExchange.gps.track('embedded_signup_form.view', location: 'question_page' ); );
$window.unbind('scroll', onScroll);
;
$window.on('scroll', onScroll);
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f213754%2freact-js-nested-nav-bar%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
it's clear and readable but i would like to point out some things :
Avoid using functions inside Render()
A function in the render method will be created each render which is a
slight performance hit. t's also messy if you put them in the render, which is a much > bigger reason
Use the child.id
instead of key
as index
when you map
through an array in render()
because Index as a key is an anti-pattern.
Keep your code as DRY as possible, the initialState can be an object outside the class instead of writing it twice or more, especially if it's a big object.
Optional : use Destructuring assignment to indicate which keys you will use in the function.
const initialState =
selected: '',
nestSelect: '',
children: [],
active: '',
class NestedNav extends Component
constructor(props)
super(props)
this.state = initialState
handleClick = title =>
this.setState( ...initialState )
mappedChildren = (child, selectedTitle) =>
const nestedParentClass = this.props
let childElement
if (child)
childElement = child.map(( id, title, children, url ) => <li
key=id
id=id
className=nestedParentClass + (this.state.nestSelect === title ? 'nest-selected' : '')
url=url><Link to='/'>title</Link>
children ?
<FontAwesomeIcon
onClick=() => this.mappedChildren(children, this.state.select)
className='i button'
icon=faArrowCircleDown /> : null
</li>)
this.setState(
selected: selectedTitle,
children: childElement,
active: 'true',
)
return ''
navListItems = data => data.map(( id, url, children, title, icon ) =>
const parentClass = this.props
return (<li
key=id
id=id
className=parentClass + (this.state.selected === title ? 'selected' : '')
url=url><i onClick=this.handleClick>icon</i><Link to='/'><span>title</span></Link>
children ?
<FontAwesomeIcon
onClick=() => this.mappedChildren(children, title)
className='i button'
icon=faArrowCircleRight /> : null
</li>)
);
render()
const data = this.props
const active = this.state.active === 'true' ? 'true' : ''
return (
<div className='nested-nav'>
<div className='container-two-' + active>
<h2>this.state.selected</h2>
this.state.children
</div>
<div className='container-one'>this.navListItems(data)</div>
</div>
)
export default NestedNav
$endgroup$
add a comment |
$begingroup$
it's clear and readable but i would like to point out some things :
Avoid using functions inside Render()
A function in the render method will be created each render which is a
slight performance hit. t's also messy if you put them in the render, which is a much > bigger reason
Use the child.id
instead of key
as index
when you map
through an array in render()
because Index as a key is an anti-pattern.
Keep your code as DRY as possible, the initialState can be an object outside the class instead of writing it twice or more, especially if it's a big object.
Optional : use Destructuring assignment to indicate which keys you will use in the function.
const initialState =
selected: '',
nestSelect: '',
children: [],
active: '',
class NestedNav extends Component
constructor(props)
super(props)
this.state = initialState
handleClick = title =>
this.setState( ...initialState )
mappedChildren = (child, selectedTitle) =>
const nestedParentClass = this.props
let childElement
if (child)
childElement = child.map(( id, title, children, url ) => <li
key=id
id=id
className=nestedParentClass + (this.state.nestSelect === title ? 'nest-selected' : '')
url=url><Link to='/'>title</Link>
children ?
<FontAwesomeIcon
onClick=() => this.mappedChildren(children, this.state.select)
className='i button'
icon=faArrowCircleDown /> : null
</li>)
this.setState(
selected: selectedTitle,
children: childElement,
active: 'true',
)
return ''
navListItems = data => data.map(( id, url, children, title, icon ) =>
const parentClass = this.props
return (<li
key=id
id=id
className=parentClass + (this.state.selected === title ? 'selected' : '')
url=url><i onClick=this.handleClick>icon</i><Link to='/'><span>title</span></Link>
children ?
<FontAwesomeIcon
onClick=() => this.mappedChildren(children, title)
className='i button'
icon=faArrowCircleRight /> : null
</li>)
);
render()
const data = this.props
const active = this.state.active === 'true' ? 'true' : ''
return (
<div className='nested-nav'>
<div className='container-two-' + active>
<h2>this.state.selected</h2>
this.state.children
</div>
<div className='container-one'>this.navListItems(data)</div>
</div>
)
export default NestedNav
$endgroup$
add a comment |
$begingroup$
it's clear and readable but i would like to point out some things :
Avoid using functions inside Render()
A function in the render method will be created each render which is a
slight performance hit. t's also messy if you put them in the render, which is a much > bigger reason
Use the child.id
instead of key
as index
when you map
through an array in render()
because Index as a key is an anti-pattern.
Keep your code as DRY as possible, the initialState can be an object outside the class instead of writing it twice or more, especially if it's a big object.
Optional : use Destructuring assignment to indicate which keys you will use in the function.
const initialState =
selected: '',
nestSelect: '',
children: [],
active: '',
class NestedNav extends Component
constructor(props)
super(props)
this.state = initialState
handleClick = title =>
this.setState( ...initialState )
mappedChildren = (child, selectedTitle) =>
const nestedParentClass = this.props
let childElement
if (child)
childElement = child.map(( id, title, children, url ) => <li
key=id
id=id
className=nestedParentClass + (this.state.nestSelect === title ? 'nest-selected' : '')
url=url><Link to='/'>title</Link>
children ?
<FontAwesomeIcon
onClick=() => this.mappedChildren(children, this.state.select)
className='i button'
icon=faArrowCircleDown /> : null
</li>)
this.setState(
selected: selectedTitle,
children: childElement,
active: 'true',
)
return ''
navListItems = data => data.map(( id, url, children, title, icon ) =>
const parentClass = this.props
return (<li
key=id
id=id
className=parentClass + (this.state.selected === title ? 'selected' : '')
url=url><i onClick=this.handleClick>icon</i><Link to='/'><span>title</span></Link>
children ?
<FontAwesomeIcon
onClick=() => this.mappedChildren(children, title)
className='i button'
icon=faArrowCircleRight /> : null
</li>)
);
render()
const data = this.props
const active = this.state.active === 'true' ? 'true' : ''
return (
<div className='nested-nav'>
<div className='container-two-' + active>
<h2>this.state.selected</h2>
this.state.children
</div>
<div className='container-one'>this.navListItems(data)</div>
</div>
)
export default NestedNav
$endgroup$
it's clear and readable but i would like to point out some things :
Avoid using functions inside Render()
A function in the render method will be created each render which is a
slight performance hit. t's also messy if you put them in the render, which is a much > bigger reason
Use the child.id
instead of key
as index
when you map
through an array in render()
because Index as a key is an anti-pattern.
Keep your code as DRY as possible, the initialState can be an object outside the class instead of writing it twice or more, especially if it's a big object.
Optional : use Destructuring assignment to indicate which keys you will use in the function.
const initialState =
selected: '',
nestSelect: '',
children: [],
active: '',
class NestedNav extends Component
constructor(props)
super(props)
this.state = initialState
handleClick = title =>
this.setState( ...initialState )
mappedChildren = (child, selectedTitle) =>
const nestedParentClass = this.props
let childElement
if (child)
childElement = child.map(( id, title, children, url ) => <li
key=id
id=id
className=nestedParentClass + (this.state.nestSelect === title ? 'nest-selected' : '')
url=url><Link to='/'>title</Link>
children ?
<FontAwesomeIcon
onClick=() => this.mappedChildren(children, this.state.select)
className='i button'
icon=faArrowCircleDown /> : null
</li>)
this.setState(
selected: selectedTitle,
children: childElement,
active: 'true',
)
return ''
navListItems = data => data.map(( id, url, children, title, icon ) =>
const parentClass = this.props
return (<li
key=id
id=id
className=parentClass + (this.state.selected === title ? 'selected' : '')
url=url><i onClick=this.handleClick>icon</i><Link to='/'><span>title</span></Link>
children ?
<FontAwesomeIcon
onClick=() => this.mappedChildren(children, title)
className='i button'
icon=faArrowCircleRight /> : null
</li>)
);
render()
const data = this.props
const active = this.state.active === 'true' ? 'true' : ''
return (
<div className='nested-nav'>
<div className='container-two-' + active>
<h2>this.state.selected</h2>
this.state.children
</div>
<div className='container-one'>this.navListItems(data)</div>
</div>
)
export default NestedNav
edited Feb 20 at 21:10
answered Feb 19 at 3:11
TakiTaki
3438
3438
add a comment |
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
var $window = $(window),
onScroll = function(e)
var $elem = $('.new-login-left'),
docViewTop = $window.scrollTop(),
docViewBottom = docViewTop + $window.height(),
elemTop = $elem.offset().top,
elemBottom = elemTop + $elem.height();
if ((docViewTop elemBottom))
StackExchange.using('gps', function() StackExchange.gps.track('embedded_signup_form.view', location: 'question_page' ); );
$window.unbind('scroll', onScroll);
;
$window.on('scroll', onScroll);
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f213754%2freact-js-nested-nav-bar%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
var $window = $(window),
onScroll = function(e)
var $elem = $('.new-login-left'),
docViewTop = $window.scrollTop(),
docViewBottom = docViewTop + $window.height(),
elemTop = $elem.offset().top,
elemBottom = elemTop + $elem.height();
if ((docViewTop elemBottom))
StackExchange.using('gps', function() StackExchange.gps.track('embedded_signup_form.view', location: 'question_page' ); );
$window.unbind('scroll', onScroll);
;
$window.on('scroll', onScroll);
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
var $window = $(window),
onScroll = function(e)
var $elem = $('.new-login-left'),
docViewTop = $window.scrollTop(),
docViewBottom = docViewTop + $window.height(),
elemTop = $elem.offset().top,
elemBottom = elemTop + $elem.height();
if ((docViewTop elemBottom))
StackExchange.using('gps', function() StackExchange.gps.track('embedded_signup_form.view', location: 'question_page' ); );
$window.unbind('scroll', onScroll);
;
$window.on('scroll', onScroll);
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
var $window = $(window),
onScroll = function(e)
var $elem = $('.new-login-left'),
docViewTop = $window.scrollTop(),
docViewBottom = docViewTop + $window.height(),
elemTop = $elem.offset().top,
elemBottom = elemTop + $elem.height();
if ((docViewTop elemBottom))
StackExchange.using('gps', function() StackExchange.gps.track('embedded_signup_form.view', location: 'question_page' ); );
$window.unbind('scroll', onScroll);
;
$window.on('scroll', onScroll);
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
njt clHNIcThZzblLx8my,pr5AFxq1iWjk45Y,G