Asp.net networking/restclient layer Planned maintenance scheduled April 23, 2019 at 23:30 UTC (7:30pm US/Eastern) Announcing the arrival of Valued Associate #679: Cesar Manara Unicorn Meta Zoo #1: Why another podcast?
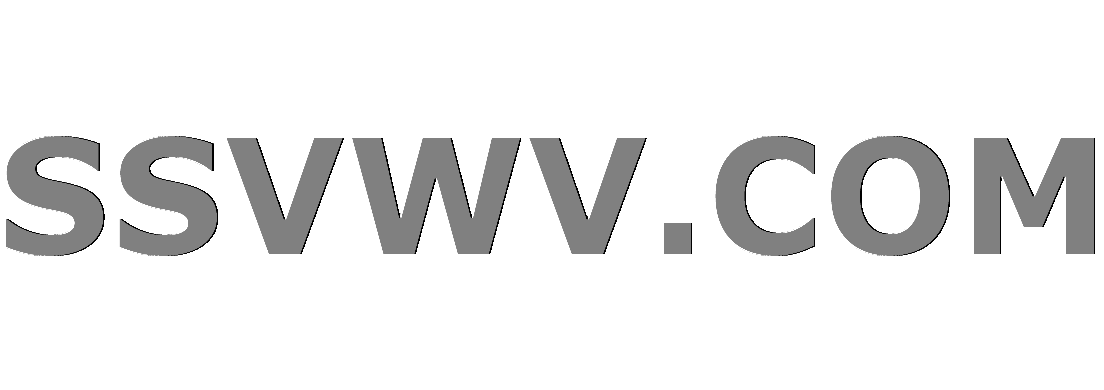
Multi tool use
How do I say "this must not happen"?
How to resize main filesystem
Weaponising the Grasp-at-a-Distance spell
How can I prevent/balance waiting and turtling as a response to cooldown mechanics
Can I cut the hair of a conjured korred with a blade made of precious material to harvest that material from the korred?
Understanding piped commands in GNU/Linux
Why does BitLocker not use RSA?
Why did Bronn offer to be Tyrion Lannister's champion in trial by combat?
How to achieve cat-like agility?
No Invitation for Tourist Visa, But i want to visit
Inverse square law not accurate for non-point masses?
How to make an animal which can only breed for a certain number of generations?
How to remove this numerical artifact?
Bash script to execute command with file from directory and condition
Sankhya yoga - Bhagvat Gita
Was the pager message from Nick Fury to Captain Marvel unnecessary?
Short story about astronauts fertilizing soil with their own bodies
Proving that any solution to the differential equation of an oscillator can be written as a sum of sinusoids.
Is it OK if I do not take the receipt in Germany?
How do you cope with tons of web fonts when copying and pasting from web pages?
Flight departed from the gate 5 min before scheduled departure time. Refund options
Why do C and C++ allow the expression (int) + 4*5?
Why is there so little support for joining EFTA in the British parliament?
Why are two-digit numbers in Jonathan Swift's "Gulliver's Travels" (1726) written in "German style"?
Asp.net networking/restclient layer
Planned maintenance scheduled April 23, 2019 at 23:30 UTC (7:30pm US/Eastern)
Announcing the arrival of Valued Associate #679: Cesar Manara
Unicorn Meta Zoo #1: Why another podcast?
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty margin-bottom:0;
$begingroup$
I have been working on creating maintainable networking layer for our Asp.Net Core Web Api project. Right now I have created Generic methods for Get, Post, Put like this.
public class BaseClient
{
private HttpClient _client;
private ILogger<BaseClient> _logger;
private string AuthToken;
public BaseClient(HttpClient client, ILogger<BaseClient> logger, AuthenticationHeader authHeader)
_client = client;
client.BaseAddress = new Uri("https://matas-test2-stag.agillic.eu/web/forward/apps/ws/");
client.DefaultRequestHeaders.Add("Accept", "application/json");
_logger = logger;
AuthToken = authHeader.AuthHeader;
public async Task<FailureResponseModel> GetFailureResponseModel(HttpResponseMessage response)
FailureResponseModel failureModel = await response.Content.ReadAsAsync<FailureResponseModel>();
failureModel.ResponseStatusCode = Convert.ToInt32(response.StatusCode);
_logger.LogError("Request Failed: Error", failureModel.ResultDetails);
return failureModel;
public async Task<object> ProcessAsync<T>(HttpRequestMessage request, NamingStrategy namingStrategy)
if (!string.IsNullOrEmpty(AuthToken))
request.Headers.Authorization = new AuthenticationHeaderValue("Bearer", AuthToken);
HttpResponseMessage response = await _client.SendAsync(request);
if (response.IsSuccessStatusCode)
_logger.LogInformation("Request Succeeded");
var dezerializerSettings = new JsonSerializerSettings
ContractResolver = new DefaultContractResolver
NamingStrategy = namingStrategy
;
T responseModel = JsonConvert.DeserializeObject<T>(await response.Content.ReadAsStringAsync(), dezerializerSettings);
return responseModel;
else
return await GetFailureResponseModel(response);
public async Task<object> GetAsync<T>(string uri)
return await GetAsync<T>(uri, new DefaultNamingStrategy());
public async Task<object> GetAsync<T>(string uri, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Get, uri))
return await ProcessAsync<T>(requestMessage, namingStrategy);
public async Task<object> PostAsync<T1, T2>(string uri, T2 content)
return await PostAsync<T1, T2>(uri, content, new DefaultNamingStrategy());
public async Task<object> PostAsync<T1, T2>(string uri, T2 content, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Post, uri))
var json = JsonConvert.SerializeObject(content);
using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json"))
requestMessage.Content = stringContent;
return await ProcessAsync<T1>(requestMessage, namingStrategy);
public async Task<object> PutAsyc<T1, T2>(string uri, T2 content)
return await PutAsyc<T1, T2>(uri, content, new DefaultNamingStrategy());
public async Task<object> PutAsyc<T1, T2>(string uri, T2 content, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Put, uri))
var json = JsonConvert.SerializeObject(content,
Formatting.None,
new JsonSerializerSettings
NullValueHandling = NullValueHandling.Ignore
);
using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json"))
requestMessage.Content = stringContent;
return await ProcessAsync<T1>(requestMessage, namingStrategy);
As it can be seen I have to write 2 Methods for Get, Post and Put due to SnakeCase and CamelCase Naming Strategies as some response consist of SnakeCase and some responses are in CamelCase
I have created ApiManager kinda class which calls above Class methods like this.
public class ClubMatasClient
private BaseClient _client;
private ILogger<ClubMatasClient> _logger;
private string AuthToken;
public ClubMatasClient(BaseClient client, ILogger<ClubMatasClient> logger, AuthenticationHeader authHeader)
_client = client;
_logger = logger;
AuthToken = authHeader.AuthHeader;
public async Task<object> GetShops(string category)
_logger.LogInformation("ClubMatas outgoing request: RequestName", nameof(GetShops));
return await _client.GetAsync<ShopsResponseModel>($"v2/shops?category=WebUtility.UrlEncode(category)");
public async Task<object> PostLogin(LoginRequestModel form)
_logger.LogInformation("ClubMatas outgoing request: RequestName", nameof(PostLogin));
return await _client.PostAsync<LoginResponseModel, LoginRequestModel>("v2/login", form, new SnakeCaseNamingStrategy());
And this class in being injected in my Controllers and I am using this ApiManager like this to call api and return response.
public async Task<ActionResult<object>> GetShops([FromQuery(Name = "category")]string category)
var response = await _httpClient.GetShops(category);
return ParseResponse<ShopsResponseModel>(response);
ParseResponse is helper Generic method which either return SuccessModel (passed as T) or failure response Model.
protected ActionResult<object> ParseResponse<T>(object response)
if (response.GetType() == typeof(T))
return Ok(response);
else
return Error(response);
Now my question is as I am fairly new to C#/ASP.net Core , is my current flow/architecture is fine, and how can I make it more elegant and more flexible.
c# asp.net-core
New contributor
Shabir jan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
I have been working on creating maintainable networking layer for our Asp.Net Core Web Api project. Right now I have created Generic methods for Get, Post, Put like this.
public class BaseClient
{
private HttpClient _client;
private ILogger<BaseClient> _logger;
private string AuthToken;
public BaseClient(HttpClient client, ILogger<BaseClient> logger, AuthenticationHeader authHeader)
_client = client;
client.BaseAddress = new Uri("https://matas-test2-stag.agillic.eu/web/forward/apps/ws/");
client.DefaultRequestHeaders.Add("Accept", "application/json");
_logger = logger;
AuthToken = authHeader.AuthHeader;
public async Task<FailureResponseModel> GetFailureResponseModel(HttpResponseMessage response)
FailureResponseModel failureModel = await response.Content.ReadAsAsync<FailureResponseModel>();
failureModel.ResponseStatusCode = Convert.ToInt32(response.StatusCode);
_logger.LogError("Request Failed: Error", failureModel.ResultDetails);
return failureModel;
public async Task<object> ProcessAsync<T>(HttpRequestMessage request, NamingStrategy namingStrategy)
if (!string.IsNullOrEmpty(AuthToken))
request.Headers.Authorization = new AuthenticationHeaderValue("Bearer", AuthToken);
HttpResponseMessage response = await _client.SendAsync(request);
if (response.IsSuccessStatusCode)
_logger.LogInformation("Request Succeeded");
var dezerializerSettings = new JsonSerializerSettings
ContractResolver = new DefaultContractResolver
NamingStrategy = namingStrategy
;
T responseModel = JsonConvert.DeserializeObject<T>(await response.Content.ReadAsStringAsync(), dezerializerSettings);
return responseModel;
else
return await GetFailureResponseModel(response);
public async Task<object> GetAsync<T>(string uri)
return await GetAsync<T>(uri, new DefaultNamingStrategy());
public async Task<object> GetAsync<T>(string uri, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Get, uri))
return await ProcessAsync<T>(requestMessage, namingStrategy);
public async Task<object> PostAsync<T1, T2>(string uri, T2 content)
return await PostAsync<T1, T2>(uri, content, new DefaultNamingStrategy());
public async Task<object> PostAsync<T1, T2>(string uri, T2 content, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Post, uri))
var json = JsonConvert.SerializeObject(content);
using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json"))
requestMessage.Content = stringContent;
return await ProcessAsync<T1>(requestMessage, namingStrategy);
public async Task<object> PutAsyc<T1, T2>(string uri, T2 content)
return await PutAsyc<T1, T2>(uri, content, new DefaultNamingStrategy());
public async Task<object> PutAsyc<T1, T2>(string uri, T2 content, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Put, uri))
var json = JsonConvert.SerializeObject(content,
Formatting.None,
new JsonSerializerSettings
NullValueHandling = NullValueHandling.Ignore
);
using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json"))
requestMessage.Content = stringContent;
return await ProcessAsync<T1>(requestMessage, namingStrategy);
As it can be seen I have to write 2 Methods for Get, Post and Put due to SnakeCase and CamelCase Naming Strategies as some response consist of SnakeCase and some responses are in CamelCase
I have created ApiManager kinda class which calls above Class methods like this.
public class ClubMatasClient
private BaseClient _client;
private ILogger<ClubMatasClient> _logger;
private string AuthToken;
public ClubMatasClient(BaseClient client, ILogger<ClubMatasClient> logger, AuthenticationHeader authHeader)
_client = client;
_logger = logger;
AuthToken = authHeader.AuthHeader;
public async Task<object> GetShops(string category)
_logger.LogInformation("ClubMatas outgoing request: RequestName", nameof(GetShops));
return await _client.GetAsync<ShopsResponseModel>($"v2/shops?category=WebUtility.UrlEncode(category)");
public async Task<object> PostLogin(LoginRequestModel form)
_logger.LogInformation("ClubMatas outgoing request: RequestName", nameof(PostLogin));
return await _client.PostAsync<LoginResponseModel, LoginRequestModel>("v2/login", form, new SnakeCaseNamingStrategy());
And this class in being injected in my Controllers and I am using this ApiManager like this to call api and return response.
public async Task<ActionResult<object>> GetShops([FromQuery(Name = "category")]string category)
var response = await _httpClient.GetShops(category);
return ParseResponse<ShopsResponseModel>(response);
ParseResponse is helper Generic method which either return SuccessModel (passed as T) or failure response Model.
protected ActionResult<object> ParseResponse<T>(object response)
if (response.GetType() == typeof(T))
return Ok(response);
else
return Error(response);
Now my question is as I am fairly new to C#/ASP.net Core , is my current flow/architecture is fine, and how can I make it more elegant and more flexible.
c# asp.net-core
New contributor
Shabir jan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
I have been working on creating maintainable networking layer for our Asp.Net Core Web Api project. Right now I have created Generic methods for Get, Post, Put like this.
public class BaseClient
{
private HttpClient _client;
private ILogger<BaseClient> _logger;
private string AuthToken;
public BaseClient(HttpClient client, ILogger<BaseClient> logger, AuthenticationHeader authHeader)
_client = client;
client.BaseAddress = new Uri("https://matas-test2-stag.agillic.eu/web/forward/apps/ws/");
client.DefaultRequestHeaders.Add("Accept", "application/json");
_logger = logger;
AuthToken = authHeader.AuthHeader;
public async Task<FailureResponseModel> GetFailureResponseModel(HttpResponseMessage response)
FailureResponseModel failureModel = await response.Content.ReadAsAsync<FailureResponseModel>();
failureModel.ResponseStatusCode = Convert.ToInt32(response.StatusCode);
_logger.LogError("Request Failed: Error", failureModel.ResultDetails);
return failureModel;
public async Task<object> ProcessAsync<T>(HttpRequestMessage request, NamingStrategy namingStrategy)
if (!string.IsNullOrEmpty(AuthToken))
request.Headers.Authorization = new AuthenticationHeaderValue("Bearer", AuthToken);
HttpResponseMessage response = await _client.SendAsync(request);
if (response.IsSuccessStatusCode)
_logger.LogInformation("Request Succeeded");
var dezerializerSettings = new JsonSerializerSettings
ContractResolver = new DefaultContractResolver
NamingStrategy = namingStrategy
;
T responseModel = JsonConvert.DeserializeObject<T>(await response.Content.ReadAsStringAsync(), dezerializerSettings);
return responseModel;
else
return await GetFailureResponseModel(response);
public async Task<object> GetAsync<T>(string uri)
return await GetAsync<T>(uri, new DefaultNamingStrategy());
public async Task<object> GetAsync<T>(string uri, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Get, uri))
return await ProcessAsync<T>(requestMessage, namingStrategy);
public async Task<object> PostAsync<T1, T2>(string uri, T2 content)
return await PostAsync<T1, T2>(uri, content, new DefaultNamingStrategy());
public async Task<object> PostAsync<T1, T2>(string uri, T2 content, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Post, uri))
var json = JsonConvert.SerializeObject(content);
using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json"))
requestMessage.Content = stringContent;
return await ProcessAsync<T1>(requestMessage, namingStrategy);
public async Task<object> PutAsyc<T1, T2>(string uri, T2 content)
return await PutAsyc<T1, T2>(uri, content, new DefaultNamingStrategy());
public async Task<object> PutAsyc<T1, T2>(string uri, T2 content, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Put, uri))
var json = JsonConvert.SerializeObject(content,
Formatting.None,
new JsonSerializerSettings
NullValueHandling = NullValueHandling.Ignore
);
using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json"))
requestMessage.Content = stringContent;
return await ProcessAsync<T1>(requestMessage, namingStrategy);
As it can be seen I have to write 2 Methods for Get, Post and Put due to SnakeCase and CamelCase Naming Strategies as some response consist of SnakeCase and some responses are in CamelCase
I have created ApiManager kinda class which calls above Class methods like this.
public class ClubMatasClient
private BaseClient _client;
private ILogger<ClubMatasClient> _logger;
private string AuthToken;
public ClubMatasClient(BaseClient client, ILogger<ClubMatasClient> logger, AuthenticationHeader authHeader)
_client = client;
_logger = logger;
AuthToken = authHeader.AuthHeader;
public async Task<object> GetShops(string category)
_logger.LogInformation("ClubMatas outgoing request: RequestName", nameof(GetShops));
return await _client.GetAsync<ShopsResponseModel>($"v2/shops?category=WebUtility.UrlEncode(category)");
public async Task<object> PostLogin(LoginRequestModel form)
_logger.LogInformation("ClubMatas outgoing request: RequestName", nameof(PostLogin));
return await _client.PostAsync<LoginResponseModel, LoginRequestModel>("v2/login", form, new SnakeCaseNamingStrategy());
And this class in being injected in my Controllers and I am using this ApiManager like this to call api and return response.
public async Task<ActionResult<object>> GetShops([FromQuery(Name = "category")]string category)
var response = await _httpClient.GetShops(category);
return ParseResponse<ShopsResponseModel>(response);
ParseResponse is helper Generic method which either return SuccessModel (passed as T) or failure response Model.
protected ActionResult<object> ParseResponse<T>(object response)
if (response.GetType() == typeof(T))
return Ok(response);
else
return Error(response);
Now my question is as I am fairly new to C#/ASP.net Core , is my current flow/architecture is fine, and how can I make it more elegant and more flexible.
c# asp.net-core
New contributor
Shabir jan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
I have been working on creating maintainable networking layer for our Asp.Net Core Web Api project. Right now I have created Generic methods for Get, Post, Put like this.
public class BaseClient
{
private HttpClient _client;
private ILogger<BaseClient> _logger;
private string AuthToken;
public BaseClient(HttpClient client, ILogger<BaseClient> logger, AuthenticationHeader authHeader)
_client = client;
client.BaseAddress = new Uri("https://matas-test2-stag.agillic.eu/web/forward/apps/ws/");
client.DefaultRequestHeaders.Add("Accept", "application/json");
_logger = logger;
AuthToken = authHeader.AuthHeader;
public async Task<FailureResponseModel> GetFailureResponseModel(HttpResponseMessage response)
FailureResponseModel failureModel = await response.Content.ReadAsAsync<FailureResponseModel>();
failureModel.ResponseStatusCode = Convert.ToInt32(response.StatusCode);
_logger.LogError("Request Failed: Error", failureModel.ResultDetails);
return failureModel;
public async Task<object> ProcessAsync<T>(HttpRequestMessage request, NamingStrategy namingStrategy)
if (!string.IsNullOrEmpty(AuthToken))
request.Headers.Authorization = new AuthenticationHeaderValue("Bearer", AuthToken);
HttpResponseMessage response = await _client.SendAsync(request);
if (response.IsSuccessStatusCode)
_logger.LogInformation("Request Succeeded");
var dezerializerSettings = new JsonSerializerSettings
ContractResolver = new DefaultContractResolver
NamingStrategy = namingStrategy
;
T responseModel = JsonConvert.DeserializeObject<T>(await response.Content.ReadAsStringAsync(), dezerializerSettings);
return responseModel;
else
return await GetFailureResponseModel(response);
public async Task<object> GetAsync<T>(string uri)
return await GetAsync<T>(uri, new DefaultNamingStrategy());
public async Task<object> GetAsync<T>(string uri, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Get, uri))
return await ProcessAsync<T>(requestMessage, namingStrategy);
public async Task<object> PostAsync<T1, T2>(string uri, T2 content)
return await PostAsync<T1, T2>(uri, content, new DefaultNamingStrategy());
public async Task<object> PostAsync<T1, T2>(string uri, T2 content, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Post, uri))
var json = JsonConvert.SerializeObject(content);
using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json"))
requestMessage.Content = stringContent;
return await ProcessAsync<T1>(requestMessage, namingStrategy);
public async Task<object> PutAsyc<T1, T2>(string uri, T2 content)
return await PutAsyc<T1, T2>(uri, content, new DefaultNamingStrategy());
public async Task<object> PutAsyc<T1, T2>(string uri, T2 content, NamingStrategy namingStrategy)
using (var requestMessage = new HttpRequestMessage(HttpMethod.Put, uri))
var json = JsonConvert.SerializeObject(content,
Formatting.None,
new JsonSerializerSettings
NullValueHandling = NullValueHandling.Ignore
);
using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json"))
requestMessage.Content = stringContent;
return await ProcessAsync<T1>(requestMessage, namingStrategy);
As it can be seen I have to write 2 Methods for Get, Post and Put due to SnakeCase and CamelCase Naming Strategies as some response consist of SnakeCase and some responses are in CamelCase
I have created ApiManager kinda class which calls above Class methods like this.
public class ClubMatasClient
private BaseClient _client;
private ILogger<ClubMatasClient> _logger;
private string AuthToken;
public ClubMatasClient(BaseClient client, ILogger<ClubMatasClient> logger, AuthenticationHeader authHeader)
_client = client;
_logger = logger;
AuthToken = authHeader.AuthHeader;
public async Task<object> GetShops(string category)
_logger.LogInformation("ClubMatas outgoing request: RequestName", nameof(GetShops));
return await _client.GetAsync<ShopsResponseModel>($"v2/shops?category=WebUtility.UrlEncode(category)");
public async Task<object> PostLogin(LoginRequestModel form)
_logger.LogInformation("ClubMatas outgoing request: RequestName", nameof(PostLogin));
return await _client.PostAsync<LoginResponseModel, LoginRequestModel>("v2/login", form, new SnakeCaseNamingStrategy());
And this class in being injected in my Controllers and I am using this ApiManager like this to call api and return response.
public async Task<ActionResult<object>> GetShops([FromQuery(Name = "category")]string category)
var response = await _httpClient.GetShops(category);
return ParseResponse<ShopsResponseModel>(response);
ParseResponse is helper Generic method which either return SuccessModel (passed as T) or failure response Model.
protected ActionResult<object> ParseResponse<T>(object response)
if (response.GetType() == typeof(T))
return Ok(response);
else
return Error(response);
Now my question is as I am fairly new to C#/ASP.net Core , is my current flow/architecture is fine, and how can I make it more elegant and more flexible.
c# asp.net-core
c# asp.net-core
New contributor
Shabir jan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Shabir jan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Shabir jan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 4 mins ago


Shabir janShabir jan
101
101
New contributor
Shabir jan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Shabir jan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Shabir jan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "196"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Shabir jan is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
var $window = $(window),
onScroll = function(e)
var $elem = $('.new-login-left'),
docViewTop = $window.scrollTop(),
docViewBottom = docViewTop + $window.height(),
elemTop = $elem.offset().top,
elemBottom = elemTop + $elem.height();
if ((docViewTop elemBottom))
StackExchange.using('gps', function() StackExchange.gps.track('embedded_signup_form.view', location: 'question_page' ); );
$window.unbind('scroll', onScroll);
;
$window.on('scroll', onScroll);
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f217883%2fasp-net-networking-restclient-layer%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Shabir jan is a new contributor. Be nice, and check out our Code of Conduct.
Shabir jan is a new contributor. Be nice, and check out our Code of Conduct.
Shabir jan is a new contributor. Be nice, and check out our Code of Conduct.
Shabir jan is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
var $window = $(window),
onScroll = function(e)
var $elem = $('.new-login-left'),
docViewTop = $window.scrollTop(),
docViewBottom = docViewTop + $window.height(),
elemTop = $elem.offset().top,
elemBottom = elemTop + $elem.height();
if ((docViewTop elemBottom))
StackExchange.using('gps', function() StackExchange.gps.track('embedded_signup_form.view', location: 'question_page' ); );
$window.unbind('scroll', onScroll);
;
$window.on('scroll', onScroll);
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f217883%2fasp-net-networking-restclient-layer%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
var $window = $(window),
onScroll = function(e)
var $elem = $('.new-login-left'),
docViewTop = $window.scrollTop(),
docViewBottom = docViewTop + $window.height(),
elemTop = $elem.offset().top,
elemBottom = elemTop + $elem.height();
if ((docViewTop elemBottom))
StackExchange.using('gps', function() StackExchange.gps.track('embedded_signup_form.view', location: 'question_page' ); );
$window.unbind('scroll', onScroll);
;
$window.on('scroll', onScroll);
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
var $window = $(window),
onScroll = function(e)
var $elem = $('.new-login-left'),
docViewTop = $window.scrollTop(),
docViewBottom = docViewTop + $window.height(),
elemTop = $elem.offset().top,
elemBottom = elemTop + $elem.height();
if ((docViewTop elemBottom))
StackExchange.using('gps', function() StackExchange.gps.track('embedded_signup_form.view', location: 'question_page' ); );
$window.unbind('scroll', onScroll);
;
$window.on('scroll', onScroll);
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
var $window = $(window),
onScroll = function(e)
var $elem = $('.new-login-left'),
docViewTop = $window.scrollTop(),
docViewBottom = docViewTop + $window.height(),
elemTop = $elem.offset().top,
elemBottom = elemTop + $elem.height();
if ((docViewTop elemBottom))
StackExchange.using('gps', function() StackExchange.gps.track('embedded_signup_form.view', location: 'question_page' ); );
$window.unbind('scroll', onScroll);
;
$window.on('scroll', onScroll);
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pKD2v 10Sl d,Z6J1Ta 5p0186kAtaxahhHiMVg9TPxvqv JMBNBsJ